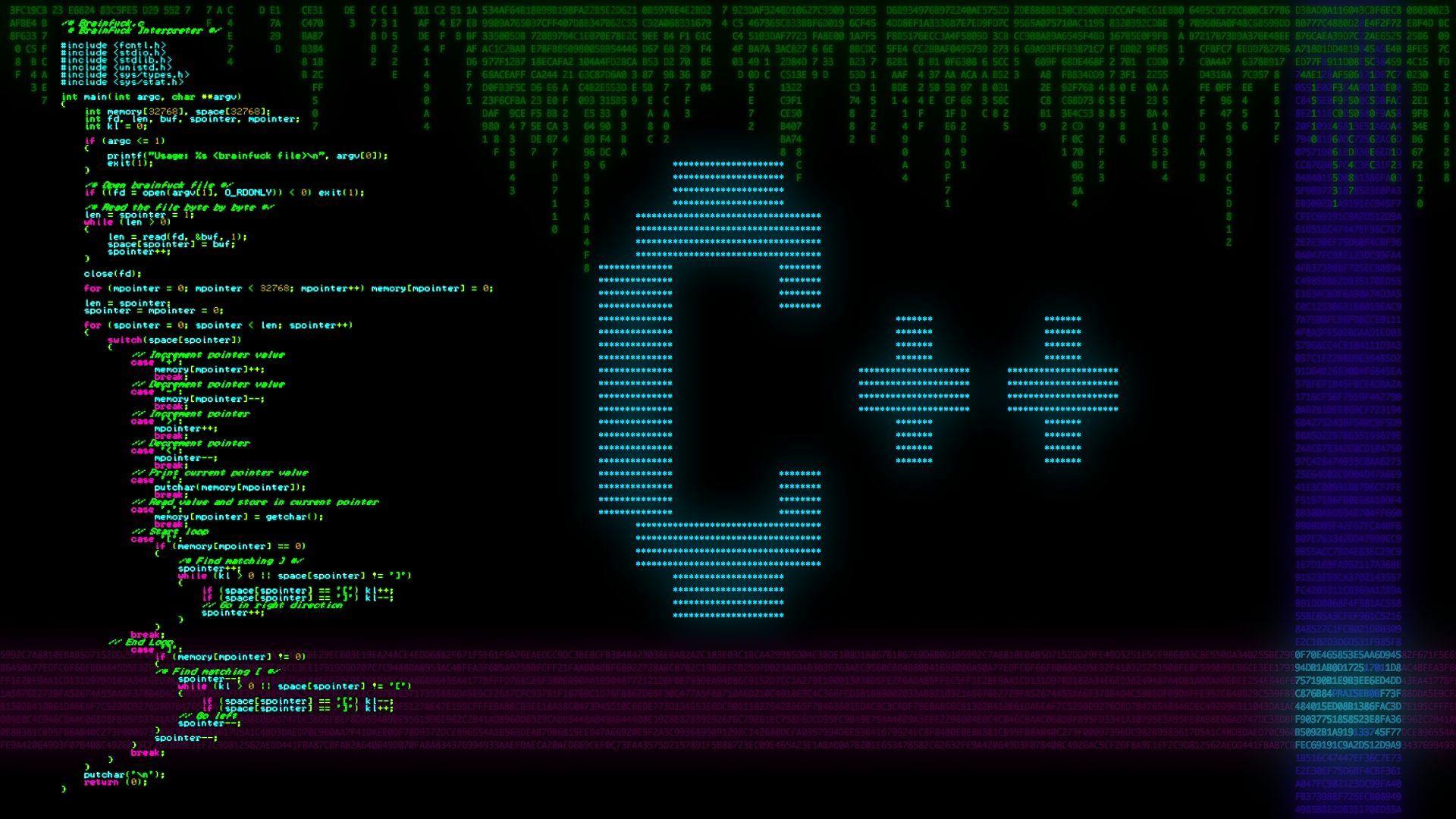
What is Member Function in C++?
A member function in C++ is a function that is defined inside a class and operates on the objects of that class. Member functions provide a way to access and manipulate the data members (also known as instance variables or attributes) of an object. A member function can also be overloaded, meaning that a class can have multiple member functions with the same name but different argument lists. Overloading member functions allows for greater flexibility and versatility in the design of a class.
A member function can be declared as either public or private. Public member functions are accessible from outside the class and can be called on objects of the class. Private member functions, on the other hand, can only be accessed within the class and are typically used to implement the internal details of a class.
Features of member functions in C++
Another feature of member functions in C++ is that they can be declared as const, which means that they promise not to modify the object on which they are called. This is useful for ensuring that objects are not modified accidentally and for allowing objects to be passed as const arguments to functions.
Additionally, member functions can also be declared as static, which means that they belong to the class rather than to individual objects. Static member functions do not have access to the non-static data members of the class and can only be called on the class itself, not on objects of the class. Learn everything about Prime Number Program in C++.
To declare a member function in a class, you use the following syntax:
Where ReturnType is the data type of the value returned by the function (or void if the function does not return a value), FunctionName is the name of the function, and ArgumentList is a comma-separated list of arguments passed to the function.
For example, the following code defines a class Rectangle with two member functions setWidth and setHeight:
To define the member functions outside of the class definition, you use the following syntax:
The :: operator is used to specify that the functions belong to the Rectangle class. The this pointer is a special pointer in C++ that refers to the current object. In the example, the this pointer is used to access the width and height data members of the Rectangle class.
Member functions can be called on objects of the class using the dot (.) operator. For example:
There are several special member functions in C++ that are automatically generated by the compiler if not defined by the programmer. These include the default constructor, copy constructor, copy assignment operator, and destructor.
The default constructor is a constructor that is called when an object is created without arguments. The default constructor sets the data members to their default values.
The copy constructor is a constructor that is called when an object is created as a copy of another object. The copy constructor performs a deep copy of the object, creating a separate and independent object.
The copy assignment operator is an operator that is called when an object is assigned to another object. The copy assignment operator performs a deep copy of the object, replacing the contents of the object being assigned to.
The destructor is a function that is called when an object is destroyed. The destructor is used to release any resources that were allocated by the object.
Finally, it's important to note that member functions can also be defined as virtual, which allows for polymorphism in C++. Polymorphism is an important feature of object-oriented programming that allows objects of different classes to be treated as objects of a common base class. This allows for greater flexibility and versatility in the design of a program. Join beginner to advanced-level online computer programming courses.
In conclusion, member functions are an important part of object-oriented programming in C++, providing a way to access and manipulate the data members of an object. Understanding member functions, and how to define and use them, is essential for effective C++ programming.