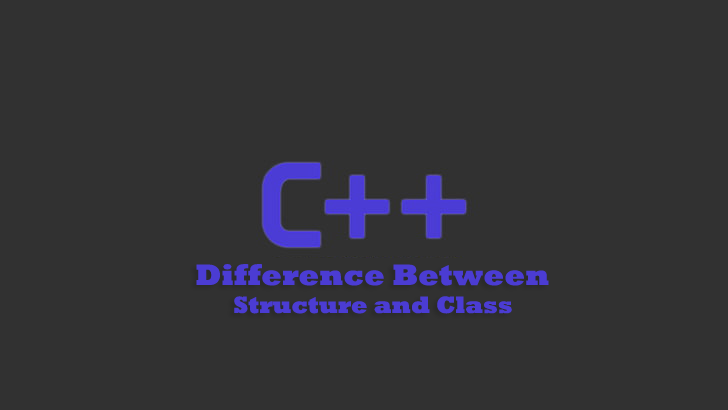
Difference Between Structure and Class in C++
In C++, both structures and classes are used to define user-defined data types, but there are some key differences between them. Here are some of the main differences between structure and class in C++:
- Default member accessibility: By default, members of a structure are public, while members of a class are private. This means that in a structure, all data members and member functions are accessible outside the structure, and in a class, they are only accessible within the class and its derived classes (if they are declared as protected).
- Inheritance: Classes support inheritance, while structures do not. This means that you can create derived classes from a class, which inherit its members and functions, but you cannot do this with a structure.
- Polymorphism: Classes support polymorphism (the ability for objects to take on different forms or have multiple behaviors), while structures do not. This is because classes can have virtual functions, which allow them to be overridden in derived classes, while structures cannot.
- Constructors and destructors: Classes can have constructors and destructors, while structures cannot. Constructors are special member functions that are called when an object is created, and are used to initialize the object's data members. Destructors are called when an object is destroyed, and are used to clean up any resources that the object has acquired.
- Syntax: The syntax for defining a class and a structure is slightly different. In C++, the keyword "class" is used to define a class, while the keyword "struct" is used to define a structure. However, aside from this keyword difference, the syntax for defining data members and member functions is identical.
- Usage: Structures are generally used for storing a collection of related data that does not require any behavior. For example, a structure might be used to represent a point in 3D space, with data members for the x, y, and z coordinates. Classes, on the other hand, are used for more complex objects that require behavior, such as a bank account class that has methods for depositing and withdrawing money.
- Size and alignment: Structures and classes can have different sizes and alignments, depending on their data members. However, there is a key difference in the default alignment of structures and classes. In C++, the default alignment for a structure is byte alignment, which means that each data member is aligned on a byte boundary. In contrast, the default alignment for a class is member alignment, which means that each data member is aligned based on its type. This can affect the memory layout of structures and classes, and can have performance implications.
- Friend functions: Friend functions are functions that are not members of a class or structure, but have access to its private and protected members. In C++, you can declare a friend function inside a class or structure to allow it to access its private members. This can be useful for creating functions that operate on a specific class or structure, but are not part of its interface. However, friend functions work slightly differently in structures and classes. In a structure, a friend function has access to all the members of the structure, including its data members and member functions. In a class, a friend function only has access to the class's public and protected members.
- Default values: In C++, you can provide default values for the data members of a class or structure. However, there is a difference in how default values are assigned. In a structure, you can initialize data members using an initializer list, or by assigning them default values directly in the data member declaration. In a class, you can only initialize data members using an initializer list in the constructor, or by assigning them default values in the constructor body.
- Namespace scope: In C++, you can define a structure or class inside a namespace. However, there is a difference in how the scope of the structure or class is affected. In a structure, the data members and member functions are automatically added to the enclosing namespace's scope. In a class, the data members and member functions are not added to the enclosing namespace's scope by default. You can use the "using" declaration or the scope resolution operator to access the class's members from the enclosing namespace.
In summary, structures and classes in C++ have some key differences in terms of member accessibility, inheritance, polymorphism, constructors and destructors, syntax, usage, size and alignment, friend functions, default values, and namespace scope. Choosing between a structure and a class depends on the specific needs of your program and how you want to represent your data and behavior. If you need to represent a simple collection of related data, a structure might be the most appropriate choice. However, if you need to create more complex objects that require behavior, such as classes that represent real-world entities, then a class would be the better choice. Learn everything about Prime Number Program in C++.